JamesNES
Well-known member
NES Maker puts all your button scripts into the static bank by default, which it really doesn't need to do 99% of the time. With a bit of messing around we can put them all into an empty bank and free up that precious space in 1F.
I'm going to use Bank 1A as that's blank from the start, but you can use any bank of course.
Part One - Moving the Input Read Script
First, in MainGameLoop.asm, we're going to find:
which is around line 42. Since we're moving our input reads to bank 1A, we just need to tell it to be in that bank first so we change it to:
Next, in LoadAllSubroutines.asm, find these two lines:
Cut and paste them straight in Bank1A.asm. In 1A by default there's some random leftover stuff you can just delete.
If you open up DataBank01_Includes.asm you'll see that it's all your input scripts. So now the code for reading inputs and the input scripts are in a new bank, we need to do few fiddly things so it doesn't keep
Now we load up Subroutines\doHandleInputReads.asm and remove a bunch of bank switches that we don't need any more (and didn't need in the first place). It looks like NES Maker was originally go to be set up to have input scripts in different banks but wasn't fully implemented.
At line 66 there's a bankswitch, we can just delete that:
Next there are three instances of:
Which have to be deleted.
And last there are several uses of ReturnBank through the file that need to be deleted.
Part Two - Fixing the Jump Script
(You can skip this if your game doesn't have jumping)
Now, if you compile and load your game it should initially appear to be all done. Left and right will work, but if you try and jump, it'll crash. This is because in the default jump script, there's a switch to Bank 1C to get info like the player's height:
These are all constant, they only change when you make changes in the character editor in NES Maker itself. So you can just replace them with numbers.
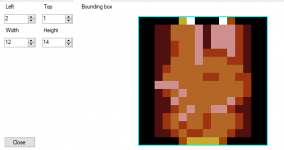
Using values from your character editor the code above becomes:
At the bottom of the script is somewhere you need to use jump speed values, these are translated from the editor differently. To find out what values you're using for that, you can open up GameEngineData\ScreenData\ObjectData\monsterObjectStartJumpSpeedLo.dat and monsterObjectStartJumpSpeedHi.dat. If your player object is your first object, their value will be the first in the tables:
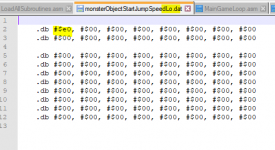
Also delete the ReturnBank at the end of the file. So with everything changed, my jump script now looks like this:
And that does it. The jump script is the only I've seen getting data from other banks, but there might be something else out there. I did this a few months ago and haven't struck any issues yet, but your mileage may vary.
I've attached a zip with the changed asm files in it for reference. I did this on a new install of NES Maker so there shouldn't be anything else changed in there.
Extra Bit
Was messing around with some stuff today (I want more than eight states), and realised you can move
out of base.asm and put it into the same bank as where you moved your input scripts to. It saves a varying amount of space depending on how many scripts you have, but for me it was like 2% of the static bank. Nothing to sniff at.
I'm going to use Bank 1A as that's blank from the start, but you can use any bank of course.
Part One - Moving the Input Read Script
First, in MainGameLoop.asm, we're going to find:
Code:
JSR doHandleInputReads
which is around line 42. Since we're moving our input reads to bank 1A, we just need to tell it to be in that bank first so we change it to:
Code:
SwitchBank #$1A
JSR doHandleInputReads
ReturnBank
Next, in LoadAllSubroutines.asm, find these two lines:
Code:
.include ROOT\Game\Subroutines\doHandleInputReads.asm
;;;further down
.include "GameData\DataBank01_Includes.asm"
Cut and paste them straight in Bank1A.asm. In 1A by default there's some random leftover stuff you can just delete.
If you open up DataBank01_Includes.asm you'll see that it's all your input scripts. So now the code for reading inputs and the input scripts are in a new bank, we need to do few fiddly things so it doesn't keep
Now we load up Subroutines\doHandleInputReads.asm and remove a bunch of bank switches that we don't need any more (and didn't need in the first place). It looks like NES Maker was originally go to be set up to have input scripts in different banks but wasn't fully implemented.
At line 66 there's a bankswitch, we can just delete that:
Code:
LDy tempBank
JSR doBankswitchY
Next there are three instances of:
Code:
LDA TargetScriptBank,y
STA temp
SwitchBank temp
Which have to be deleted.
And last there are several uses of ReturnBank through the file that need to be deleted.
Part Two - Fixing the Jump Script
(You can skip this if your game doesn't have jumping)
Now, if you compile and load your game it should initially appear to be all done. Left and right will work, but if you try and jump, it'll crash. This is because in the default jump script, there's a switch to Bank 1C to get info like the player's height:
Code:
SwitchBank #$1C
LDY Object_type,x
LDA ObjectBboxTop,y
CLC
ADC ObjectHeight,y
sta temp2
LDA Object_x_hi,x
CLC
ADC ObjectBboxLeft,y
STA temp
JSR getPointColTable
;;there are more further down the script too
These are all constant, they only change when you make changes in the character editor in NES Maker itself. So you can just replace them with numbers.
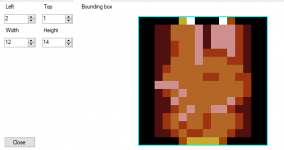
Using values from your character editor the code above becomes:
Code:
LDA #$0F;; top + height
sta temp2
LDA Object_x_hi,x
CLC
ADC #$02
STA temp
JSR getPointColTable
At the bottom of the script is somewhere you need to use jump speed values, these are translated from the editor differently. To find out what values you're using for that, you can open up GameEngineData\ScreenData\ObjectData\monsterObjectStartJumpSpeedLo.dat and monsterObjectStartJumpSpeedHi.dat. If your player object is your first object, their value will be the first in the tables:
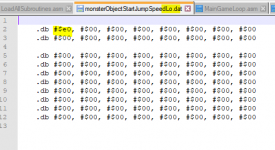
Also delete the ReturnBank at the end of the file. So with everything changed, my jump script now looks like this:
Code:
;;;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;; Only can jump if the place below feet is free.
LDA #$0F
sta temp2
LDA Object_x_hi,x
CLC
ADC #$02
STA temp
JSR getPointColTable
LDA Object_y_hi,x
CLC
ADC #$02
CLC
ADC temp2
STA temp1
CheckCollisionPoint temp, temp1, #$01, tempA ;; check below feet to see if it is solid.
;;; if it is (equal), can jump.
;;; if not, skips jumping.
BNE +checkMore
JMP +doJump
+checkMore
CheckCollisionPoint temp, temp1, #$07, tempA ;; check below feet to see if it is jumpthrough platform.
;;; if it is (equal), can jump.
;;; if not, skips jumping.
BNE +checkMore
JMP +doJump
+checkMore
CheckCollisionPoint temp, temp1, #$09, tempA ;; check below feet to see if it is prize block .
;;; if it is (equal), can jump.
;;; if not, skips jumping.
BNE +checkMore
JMP +doJump
+checkMore
CheckCollisionPoint temp, temp1, #$0A, tempA ;; check below feet to see if it is ladder.
;;; if it is (equal), can jump.
;;; if not, skips jumping.
BNE +dontDoJump
JMP +doJump
+dontDoJump
;; check second point.
LDA #$0C
CLC
ADC temp
STA temp
JSR getPointColTable
CheckCollisionPoint temp, temp1, #$01, tempA ;; check below feet to see if it is solid.
;;; if it is (equal), can jump.
;;; if not, skips jumping.
BEQ +doJump
CheckCollisionPoint temp, temp1, #$07, tempA ;; check below feet to see if it is jumpthrough platform.
;;; if it is (equal), can jump.
;;; if not, skips jumping.
BEQ +doJump
CheckCollisionPoint temp, temp1, #$09, tempA ;; check below feet to see if it is jumpthrough platform.
;;; if it is (equal), can jump.
;;; if not, skips jumping.
BEQ +doJump
CheckCollisionPoint temp, temp1, #$0A, tempA ;; check below feet to see if it is ladder.
;;; if it is (equal), can jump.
;;; if not, skips jumping.
BEQ +doJump
JMP +skipJumping
+doJump:
TXA
STA temp ;; assumes the object we want to move is in x.
PlaySound #sfx_thump
LDA #$E0
EOR #$FF
STA Object_v_speed_lo,x
LDA #$03
EOR #$FF
STA Object_v_speed_hi,x
TXA
STA temp ;; assumes the object we want to move is in x.
; change the object's action so that he is in jump mode.
+skipJumping:
RTS
And that does it. The jump script is the only I've seen getting data from other banks, but there might be something else out there. I did this a few months ago and haven't struck any issues yet, but your mileage may vary.
I've attached a zip with the changed asm files in it for reference. I did this on a new install of NES Maker so there shouldn't be anything else changed in there.
Extra Bit
Was messing around with some stuff today (I want more than eight states), and realised you can move
Code:
.include "GameData\ScriptTables.asm"
out of base.asm and put it into the same bank as where you moved your input scripts to. It saves a varying amount of space depending on how many scripts you have, but for me it was like 2% of the static bank. Nothing to sniff at.
Attachments
Last edited: