m8si
Active member
Found out an easy way to hide an object behind a tile during a specific action step.
In your Subroutines/doDrawSprites.asm find this spot and change the code. In my project it was at line 266
Then make an action step flag "Hide Behind". Note that it has to be Flag 5 or otherwise the code is not going to work.
And that's it. Now just tick that "Hide Behind" for the Action Steps you wish to hide the object behind tiles.
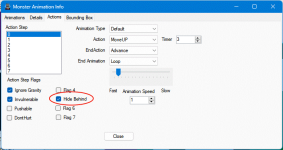
Advanced: if you for some reason need to use other place than "Flag 5" you can always shift the position of the bit with LSR / ASL. Just make sure that you shift the bit to the correct position before AND #%00100000. That is the place of the bit that holds sprite priority. (foreground/background)
Flag 6 for example:
In your Subroutines/doDrawSprites.asm find this spot and change the code. In my project it was at line 266
Code:
doDrawSpritesLoop:
LDA (temp16),y
clc
ADC temp3
STA tempC ;; the calculated table position, with offest.
;; tempC becomes the "tile to draw".
INY
LDA (temp16),y
STA tempD ;; the next value is the attribute to draw.
INY ;; increasing again sets us up for the next sprite.
;; now we can use tempA-D to draw our sprite using the macro.
;; MOVE TO BACK m8si **************************************
LDA Object_vulnerability,x
AND #%00100000
ORA tempD
STA tempD
;; MOVE TO BACK m8si **************************************
;;;;;;;;;;;; Here, we need to evaluate if this tile should be drawn
;;;;;;;;;;;; based on the comparison to camX.
JSR evaluateTileAgainstCameraPosition
BEQ thisTileIsInCamRange
JMP thisTileIsOffScreen
thisTileIsInCamRange
DrawSprite tempA, tempB, tempC,tempD
Then make an action step flag "Hide Behind". Note that it has to be Flag 5 or otherwise the code is not going to work.
And that's it. Now just tick that "Hide Behind" for the Action Steps you wish to hide the object behind tiles.
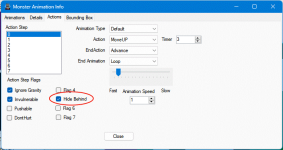
Advanced: if you for some reason need to use other place than "Flag 5" you can always shift the position of the bit with LSR / ASL. Just make sure that you shift the bit to the correct position before AND #%00100000. That is the place of the bit that holds sprite priority. (foreground/background)
Flag 6 for example:
Code:
LDA Object_vulnerability,x
LSR ;;; Action step is #%01000000 if "Flag 6 is ticked" Shift one to the right so it becomes #%00100000
AND #%00100000
ORA tempD
STA tempD