baardbi
Well-known member
There is a tutorial by 9Panzer here on the forum, but I thought I would add another on how to make a simple breakable tile. This script adds an explosion when the tile breaks as well as letting you choose if you want every player weapon to break the tile or only a specific weapon. This script was made for the MetroVania module but it should work fine for most other modules as well.
1. Put the following code in a blank file and save it as BreakableTile.asm. Put the file in a folder that makes sense to you. You could for example put it in the default Tile folder: GameEngineData\Routines\BASE_4_5\Game\TileScripts
LDA Object_flags,x
AND #%00000100 ;Player weapon
BNE +doBreakThisTile
JMP +skipBreakThisTile
Then uncomment the following lines:
LDA Object_type,x
CMP #1 ;Player projectile (game object 1)
BEQ +doBreakThisTile
JMP +skipBreakThisTile
This script uses game object 8 as the explosion animation when the tile breaks. This can easily be changed at the line CreateObjectOnScreen...
Also make sure that the tile graphic you want to use is set correctly at the line ChangeTileAtCollision... The metatile number is not the same as the tile number. We count these in 8x8 pixel blocks. Use this picture to find the top left corner of your tile graphic for the broken tile:
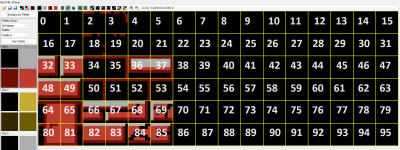
2. Assign the new BreakableTile.asm file to a free tile number. You do this in Project Settings - Script Settings:
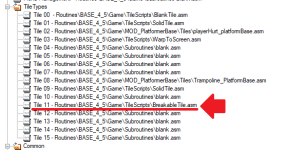
3. Rename the tile in Project Settings - Project Labels - Tile Types:
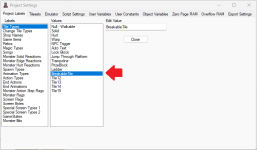
4. That's it. Now you have a breakable tile with an explosion effect.
1. Put the following code in a blank file and save it as BreakableTile.asm. Put the file in a folder that makes sense to you. You could for example put it in the default Tile folder: GameEngineData\Routines\BASE_4_5\Game\TileScripts
PS! By default this script will break the tile with any player weapon. If you want a specific weapon (game object) to break tiles you need to remove these lines at the top:;;; Breakable tile
LDA Object_flags,x
AND #%00000100 ;Player weapon
BNE +doBreakThisTile
JMP +skipBreakThisTile
; LDA Object_type,x
; CMP #1 ;Player projectile (game object 1)
; BEQ +doBreakThisTile
; JMP +skipBreakThisTile
+doBreakThisTile:
LDA updateScreenData
AND #%0000100
BEQ +doTile
RTS
+doTile
LDA tileX
AND #%11110000
LSR
LSR
LSR
LSR
STA tempA
LDA tileY
AND #%11110000
STA tempB
LDA Object_screen,x
STA tempC
DestroyObject ;Destroy player weapon
CreateObjectOnScreen tempA, tempB, #8, #0, tempC ;Create explosion (game object 8)
;; arg0 = x
;; arg1 = y
;; arg2 = object
;; arg3 = beginning state
;; arg4 = what screen do youw ant to create this object on?
ChangeTileAtCollision #2, #0 ; Metatile: Tile graphic at position 2 Collision: NULL (0)
; arg0 = metatile to change into
; arg1 = collision to change into.
JSR doWaitFrame
JMP +doneBreakableTile
+skipBreakThisTile:
LDA ObjectUpdateByte
ORA #%00000001
STA ObjectUpdateByte ;; makes solid
+doneBreakableTile:
LDA Object_flags,x
AND #%00000100 ;Player weapon
BNE +doBreakThisTile
JMP +skipBreakThisTile
Then uncomment the following lines:
LDA Object_type,x
CMP #1 ;Player projectile (game object 1)
BEQ +doBreakThisTile
JMP +skipBreakThisTile
This script uses game object 8 as the explosion animation when the tile breaks. This can easily be changed at the line CreateObjectOnScreen...
Also make sure that the tile graphic you want to use is set correctly at the line ChangeTileAtCollision... The metatile number is not the same as the tile number. We count these in 8x8 pixel blocks. Use this picture to find the top left corner of your tile graphic for the broken tile:
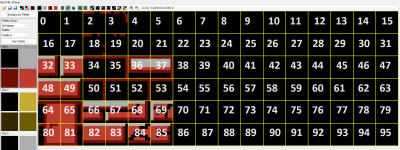
2. Assign the new BreakableTile.asm file to a free tile number. You do this in Project Settings - Script Settings:
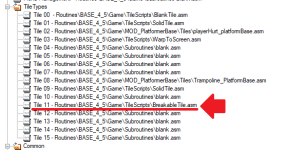
3. Rename the tile in Project Settings - Project Labels - Tile Types:
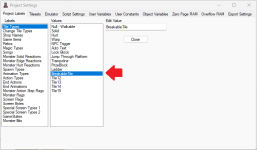
4. That's it. Now you have a breakable tile with an explosion effect.
Last edited: