baardbi
Well-known member
This will display the health of the enemy you're currently attacking in the sprite HUD. Since this tutorial is based on the sprite HUD, enemies can't have more than 8 health. This is because each health unit is a sprite and the NES can only show 8 sprites on a horizontal line.
This tutorial also includes a monster death animation (monster death object) in the hurtMonster script. You need to add an object for this (object 15 in this example) or modify this according to your game.

Before you begin I recommend you backup the following two scripts:
- doDrawSpriteHud_Brawler.asm
- hurtMonster_BrawlerBase.asm
OK. Here we go:
1. Make two new User Variables: enemyHealthTemp and enemyHealthMaxTemp
2. If you haven't set up a sprite HUD yet, then you can copy this into you doDrawSpriteHud_Brawler.asm.
If you already have a sprite HUD script set up you just need to add this below your other DrawSpriteHud line:
In this example I use tile 125 and 126 in the GameObjectTiles.bmp file to show full and empty tiles. You need to adjust these values according to your game graphics.
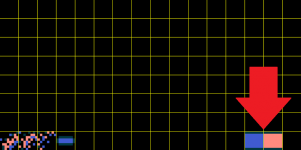
3. If you haven't done any changes to the hurtMonster_BrawlerBase.asm script you can replace it with the following code and you're done:
PS!!! Note that this script includes sound effects. Please change or remove these lines (PlaySound...) according to your game.
If you already have your own modified hurtMonster script you need to add these changes to it:
- After this line "ChangeActionStep temp, #$07" (around line 15)
Add this:
- After this part (somewhere around line 25):
BMI +healthBelowZero
STA Object_health,x
Add this:
- After the line DestroyObject (somewhere around line 45):
STA tempD
DestroyObject
Add this:
That's it. You should now have an enemy health bar in your sprite HUD when you attack enemies.
Please notify me if you find an error in this tutorial or if something is missing.
This tutorial also includes a monster death animation (monster death object) in the hurtMonster script. You need to add an object for this (object 15 in this example) or modify this according to your game.

Before you begin I recommend you backup the following two scripts:
- doDrawSpriteHud_Brawler.asm
- hurtMonster_BrawlerBase.asm
OK. Here we go:
1. Make two new User Variables: enemyHealthTemp and enemyHealthMaxTemp
2. If you haven't set up a sprite HUD yet, then you can copy this into you doDrawSpriteHud_Brawler.asm.
;;; Here is an example of how to do a sprite hud.
;;; Arg5, the one that has the value of myVar, must correspond to a user variable you have in your game.
;;; Don't forget, you can only draw 8 sprites per scanline, so a sprite hud can only be 8 sprites wide max.
LDA gameState
BEQ +drawSpriteHud
JMP +dontDrawSpriteHud
+drawSpriteHud:
DrawSpriteHud #16, #16, #125, myMaxHealth, #126, myHealth, #%00000000 ;;;; this draws health
; arg0 = starting position in pixels, x
; arg1 = starting position in pixels, y
; arg2 = sprite to draw, CONTAINER
; arg3 = MAX
; arg4 = sprite to draw, FILLED
; arg5 = variable.
; arg6 = attribute
;;;; Draw enemy health
LDA enemyHealthTemp
BNE +drawEnemyHealth
JMP +dontDrawSpriteHud
+drawEnemyHealth:
DrawSpriteHud #16, #28, #125, enemyHealthMaxTemp, #126, enemyHealthTemp, #%00000010 ;;;; this draws enemy health
+dontDrawSpriteHud:
If you already have a sprite HUD script set up you just need to add this below your other DrawSpriteHud line:
;;;; Draw enemy health
LDA enemyHealthTemp
BNE +drawEnemyHealth
JMP +dontDrawSpriteHud
+drawEnemyHealth:
DrawSpriteHud #16, #28, #125, enemyHealthMaxTemp, #126, enemyHealthTemp, #%00000010 ;;;; this draws enemy health
In this example I use tile 125 and 126 in the GameObjectTiles.bmp file to show full and empty tiles. You need to adjust these values according to your game graphics.
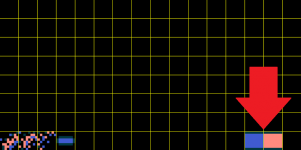
3. If you haven't done any changes to the hurtMonster_BrawlerBase.asm script you can replace it with the following code and you're done:
PS!!! Note that this script includes sound effects. Please change or remove these lines (PlaySound...) according to your game.
LDA gameHandler
AND #%10000000
BEQ +canHurtPlayer
JMP +skipHurt
+canHurtPlayer:
TXA
STA temp
GetActionStep temp
CMP #$07 ;; hurt state.
BNE +notAlreadyInHurtState
JMP +skipHurt
+notAlreadyInHurtState
PlaySound #sfx_flick
ChangeActionStep temp, #$07
;;; Load enemy max health to temp variable (used for sprite hud)
LDY Object_type,x ;;; baardbi
LDA ObjectHealth,y ;;; baardbi
STA enemyHealthMaxTemp ;;; baardbi
LDA Object_health,x
SEC
SBC #$01
BEQ +healthBelowZero
BMI +healthBelowZero
STA Object_health,x
;;; Update enemy health temp variable (used for sprite hud)
STA enemyHealthTemp ;;; baardbi
JMP +skipHurt
+healthBelowZero
LDA Object_x_hi,x
CLC
ADC #$04
STA tempA
LDA Object_y_hi,x
CLC
ADC #$04
STA tempB
LDA Object_screen,x
ADC #$00
STA tempD
DestroyObject
;;; When enemy is destroyed set temp variable to 0
LDA #$00 ;;; baardbi
STA enemyHealthTemp ;;; baardbi
CreateObjectOnScreen tempA, tempB, #$0F, #$00, tempD ;;; Create enemy death animation
;; arg0 = x
;; arg1 = y
;; arg2 = object
;; arg3 = beginning state
;; arg4 = what screen do youw ant to create this object on?
PlaySound #sfx_kerchunk
;;;; if this is set to a right edge
;;;; flip the bit so that scrolling can continue
;;;; if all bad guys are gone.
CountObjects #%00001000
BNE +notZeroCount
LDA scrollByte
ORA #%00000010
STA scrollByte
;;; if there are no more monsters left, we want to disable
;;; the edge check for scrolling.
LDA ScreenFlags00
AND #%11101111
STA ScreenFlags00
+notZeroCount:
+skipHurt
If you already have your own modified hurtMonster script you need to add these changes to it:
- After this line "ChangeActionStep temp, #$07" (around line 15)
Add this:
;;; Load enemy max health to temp variable (used for sprite hud)
LDY Object_type,x ;;; baardbi
LDA ObjectHealth,y ;;; baardbi
STA enemyHealthMaxTemp ;;; baardbi
- After this part (somewhere around line 25):
BMI +healthBelowZero
STA Object_health,x
Add this:
;;; Update enemy health temp variable (used for sprite hud)
STA enemyHealthTemp ;;; baardbi
- After the line DestroyObject (somewhere around line 45):
STA tempD
DestroyObject
Add this:
;;; When enemy is destroyed set temp variable to 0
LDA #$00 ;;; baardbi
STA enemyHealthTemp ;;; baardbi
CreateObjectOnScreen tempA, tempB, #$0F, #$00, tempD ;;; Create enemy death animation
That's it. You should now have an enemy health bar in your sprite HUD when you attack enemies.
Please notify me if you find an error in this tutorial or if something is missing.