baardbi
Well-known member
I recently published a tutorial on how to fade out screens, but here's one that both fades in and out. It fades in and out both the background and the sprites.
PS! This will take up 25 bytes of RAM.
Before you begin make sure you do the following:
- Set up user screen bytes:
www.nesmakers.com
- Create a Zero Page variable called backgroundPaletteBackup and make sure it is set up with 12 bytes
- Create a Zero Page variable called spritePaletteBackup and make sure it is set up with 12 bytes
- Create a User Variable called screenFadeIn
- Download the attached file called fading.zip. Unzip it and place the file fading.asm in ...\GameEngineData\Routines\BASE_4_5\Game\Subroutines
1. Go to Project Settings - Script Settings. At the top (under System) you will find a script define called Load Subroutines. Select it and click Edit. Put this following line at the top of the file:
2. Go to Project Settings - Project Labels and find Screen Flags. Change Screen Flag 7 to Fade In.
3. Put the following code in the extraScreenLoad file. It's in Project Settings - Script Settings. You can find it under Game. Select Extra Screen Load and click Edit.
4. Put the following code at the end of the doSpritePostDraw file. It's in Project Settings - Script Settings. You can find it under Game. Select Sprite Post-draw and click Edit.
5. Set all colors in background palette 63 to black. Do the same for monster palette 63.
Now you're done. On the screens that you want to fade in you just select Fade In under Screen Info.
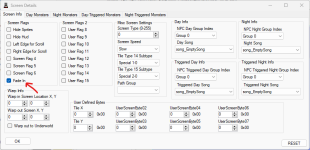
You can use fade in and fade out wherever you want in your code by writing:
or
For example you can make your warp tiles fade the screen to black before a warp by changing your warp tile to this:
The only thing I have noticed is that when fading in to a new screen the monsters and game objects are not faded in (only the player). They will pop in after the fade is complete.
PS! This will take up 25 bytes of RAM.
Before you begin make sure you do the following:
- Set up user screen bytes:
Making userScreenByte0-7 work! (Plus Bonus!)
Hey guys, got a quick code edit which will actually link those userScreenBytes on your Screen Info options! Why is this useful? Well, you can store a number in any of those 8 boxes, that is unique to the screen, and it will show up in a variable in game. This can give you some powerful...
- Create a Zero Page variable called backgroundPaletteBackup and make sure it is set up with 12 bytes
- Create a Zero Page variable called spritePaletteBackup and make sure it is set up with 12 bytes
- Create a User Variable called screenFadeIn
- Download the attached file called fading.zip. Unzip it and place the file fading.asm in ...\GameEngineData\Routines\BASE_4_5\Game\Subroutines
1. Go to Project Settings - Script Settings. At the top (under System) you will find a script define called Load Subroutines. Select it and click Edit. Put this following line at the top of the file:
Save the file and close it..include ROOT\Game\Subroutines\fading.asm
2. Go to Project Settings - Project Labels and find Screen Flags. Change Screen Flag 7 to Fade In.
3. Put the following code in the extraScreenLoad file. It's in Project Settings - Script Settings. You can find it under Game. Select Extra Screen Load and click Edit.
Put it right above doneWithExtraCheck: Save the file and close it.LDA ScreenFlags00
AND #%00000001
BEQ dontFadeIn
LDA #1
STA screenFadeIn
dontFadeIn:
4. Put the following code at the end of the doSpritePostDraw file. It's in Project Settings - Script Settings. You can find it under Game. Select Sprite Post-draw and click Edit.
Put it at the bottom of the file. Save the file and close it.;;; Handle fade in
LDA screenFadeIn
BEQ +dontFadeIn
JSR fadeIn
LDA #0
STA screenFadeIn
+dontFadeIn:
5. Set all colors in background palette 63 to black. Do the same for monster palette 63.
Now you're done. On the screens that you want to fade in you just select Fade In under Screen Info.
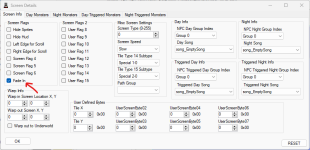
You can use fade in and fade out wherever you want in your code by writing:
JSR fadeIn
or
JSR fadeOut
For example you can make your warp tiles fade the screen to black before a warp by changing your warp tile to this:
Or you can change the input script startGameWithNewContinuePoints to fade out the screen when you press the start button on the start screen:CPX player1_object
BNE notPlayerForWarpTile
JSR fadeOut
WarpToScreen warpToMap, warpToScreen, #$01
;; arg0 = warp to map. 0= map1. 1= map2.
;; arg1 = screen to warp to.
;; arg2 = screen transition type - most likely use 1 here.
;; 1 = warp, where it observes the warp in position for the player.
notPlayerForWarpTile:
;;; This script can be used as a start game input script.
;;; It will take you to the first screen and set up new continue points based on
;;; that screen's info.
JSR fadeOut
LDA screenUpdateByte
ORA #%00000100
STA screenUpdateByte
LDA warpToMap
STA warpMap
LDA warpToScreen
STA currentNametable
LDX player1_object
STA Object_screen,x
LDA #$01
STA screenTransitionType ;; is of warp type
LDA gameHandler
ORA #%10000000
STA gameHandler ;; this will set the next game loop to update the screen.
RTS
The only thing I have noticed is that when fading in to a new screen the monsters and game objects are not faded in (only the player). They will pop in after the fade is complete.
Attachments
Last edited: