TakuikaNinja
Active member
Man, I wish I was joking.
Here is a replacement for doGetRandomNumber to get a higher quality RNG sequence with more efficient code!
Source: An tiny, fast, 8-bit pseudo-random number generator in 6502 assembly
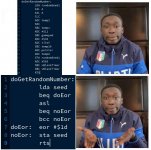
Here is a replacement for doGetRandomNumber to get a higher quality RNG sequence with more efficient code!
Source: An tiny, fast, 8-bit pseudo-random number generator in 6502 assembly
Code:
doGetRandomNumber:
lda randomSeed1
beq +doEor
asl
beq +noEor
bcc +noEor
+doEor: eor #$1d
+noEor: sta randomSeed1
rts
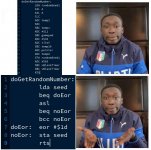
Last edited: