baardbi
Well-known member
Thanks to dale coop for a fix to this code!
Ever since the first NESmaker I have not been completely happy with how ladders are handled in platform games. I want my character to stop moving when I release the D-pad. I also want my player to snap to the ladder, so that he doesn't climb up the very left or right edge of the ladder. As far as I know this should work in any of the platform modules.
Here's what I did:
1. In the middle of the input scripts climbLadderUp and climbLadderDown put the following code after these lines:
;; there is a ladder under my feet..
LDA Object_y_hi,x
CLC
ADC #$01 ;; ladder speed
STA Object_y_hi,x
LDA tileX
AND #%11110000
;; Add or subtract player offset here if
;; you need to adjust the player position
;;
;; -- Subtract offset:
; SEC
; SBC #0 ;; Player x position
;
;; -- Or add offset:
; CLC
; ADC #0 ;; Player x position
;;
STA Object_x_hi,x
Your player will now snap to the ladder perfectly every time.
So now for the animation part. You need to have two action states for your player reserved for climbing. One for the climb animation and one for "idle climb". Use action state 3 for the actual climb animation and action state 4 for the "idle climb" (you can use one of the climb frames or make your own idle climb frame).
2. In the input script changeActionToStop change the part under changeToStop_NoDpadPressed to this:
changeToStop_NoDpadPressed:
GetActionStep temp
CMP #$03 ; Are we climbing?
BNE normalIdle
ChangeActionStep temp, #$04 ;; assumes that "climb idle" is in action 4
JMP doneChangeToStop
normalIdle:
ChangeActionStep temp, #$00 ;; assumes that "idle" is in action 0
doneChangeToStop:
RTS
3. In the Input Editor you need to add the changeActionToStop script to "release UP" and "release DOWN" on the D-pad. It should look like this:
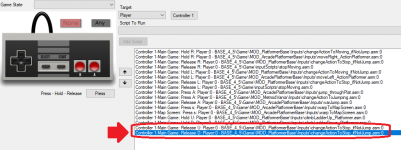
That's it! Your player should now snap to the ladders and stop the animation when the D-pad is released.
Let me know if I missed something or if I made a mistake when writing this tutorial.
Attachments
Last edited: